For the database setup, please refer to http://blog.kerul.net/2014/04/insert-new-record-on-android-online.html
INTRODUCTION
This tutorial will demonstrate how to retrieve an records form an online database. We are using the JSON data format to transfer the database content to the Android client.
STEP 1: JSON DATA FORMAT GENERATOR (server-side)
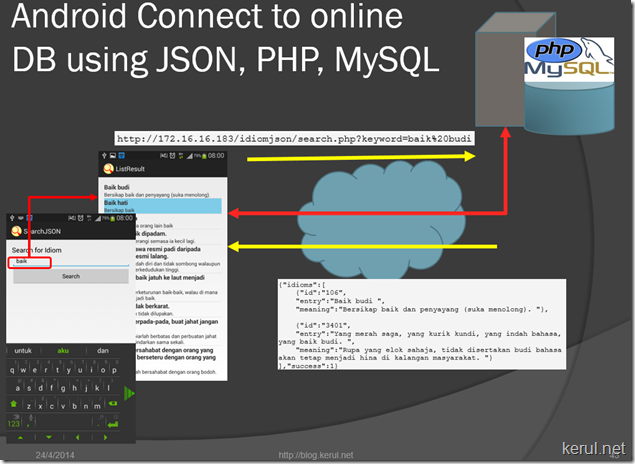
Overview illustration (click to enlarge)
In order to fetch records form the database, we will use the JSON data format generator using the PHP script. Add this file named search.php to the existing folder in the idiomjson folder, in htdocs. (Refer STEP 1 of this article http://blog.kerul.net/2014/04/insert-new-record-on-android-online.html)
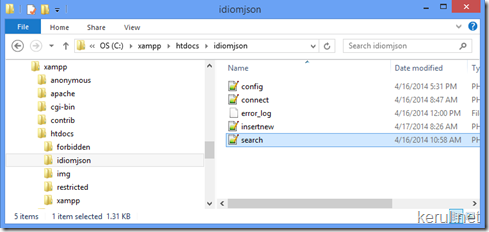
search.php
When the page is called directly from the browser, this is the JSON formatted data you will see.
STEP 2: CREATE A NEW PROJECT IN ECLIPSE-ADT (Android client-side)
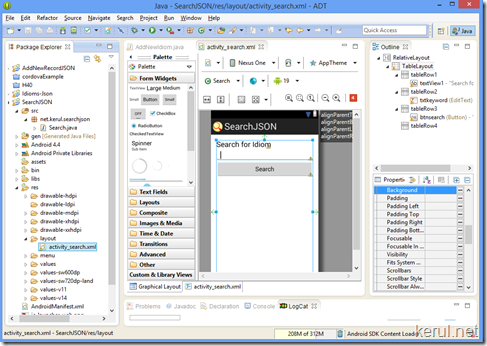
The UI consist of an EditText with id txtkeyword, and a Button id btnsearch.
This is the UI XML file
Search.java
This is only has the UI of the keyword entry. It will be calling another page/screen which will list the idioms that match the search keyword.
Create the ListView named ListResult activity to handle the result listing.
To create a new activity, right click on project and choose New –> Other…
Use the name ListResult for the new activity.
inside this UI XML file copy paste the following XML code. It must have ListView with the id named list.
activity_list_result.xml
Then add another XML file in the layout folder, by right clicking on the layout folder->New->Android XML File. This is to show the record. It will be reiterated for all records successfully fetched. It must be named list_view.xml; it will be called by activity_list_result.xml to populate the idiom data.
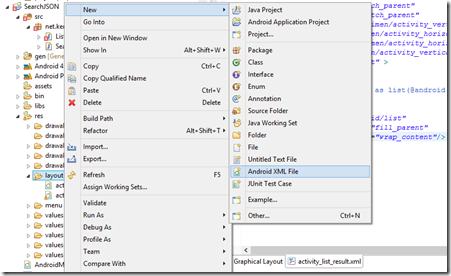
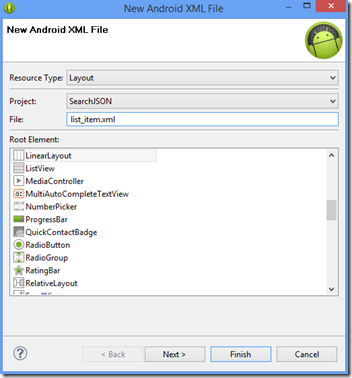
list_view.xml
and the ListResult.java file to do the fetch record and display processes.
ListResult.java
AND don’t forget the JSONParser.java file. Right click on the package name –>New->Class
JSONParser.java
AndroidManifest.xml – don’t forget to add INTERNET Uses Permission. And save the file.
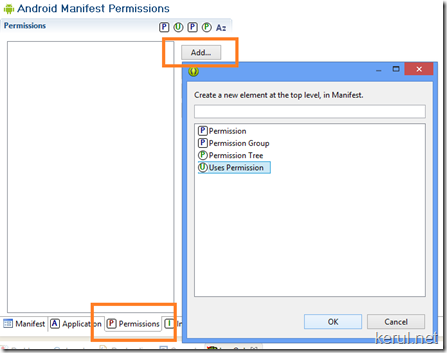
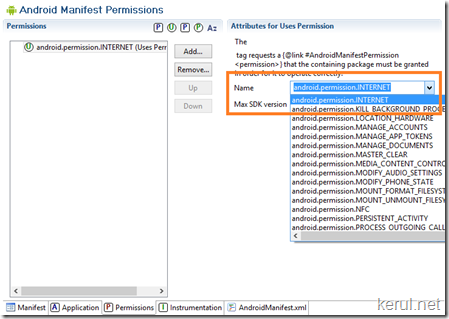
Run on your device/emulator… All the best
.
INTRODUCTION
This tutorial will demonstrate how to retrieve an records form an online database. We are using the JSON data format to transfer the database content to the Android client.
Overview illustration (click to enlarge)
In order to fetch records form the database, we will use the JSON data format generator using the PHP script. Add this file named search.php to the existing folder in the idiomjson folder, in htdocs. (Refer STEP 1 of this article http://blog.kerul.net/2014/04/insert-new-record-on-android-online.html)
search.php
<?php //search.php /* * Following code will search idiom based on keywords */ // array for JSON response $response = array(); // include connect class require_once __DIR__ . '/connect.php'; // connecting to db $db = new DB_CONNECT(); $keyword=$_GET["keyword"]; // get idioms based on keyword /* $result = mysql_query("SELECT * FROM a_senarai_e WHERE " . "MATCH(entri) AGAINST ('$keyword' ". "IN NATURAL LANGUAGE MODE)") or die(mysql_error()); */ //using LIKE $result = mysql_query("SELECT * FROM idiomlist WHERE entry LIKE '%$keyword%' LIMIT 0, 20") or die(mysql_error()); // check for empty result if (mysql_num_rows($result) > 0) { // looping through all results $response["idioms"] = array(); while ($row = mysql_fetch_array($result)) { // temp user array $idioms= array(); $idioms["id"] = $row["id"]; $idioms["entry"] = $row["entry"]; $idioms["meaning"] = $row["meaning"]; // push single idiom array into final response array array_push($response["idioms"], $idioms); } // success $response["success"] = 1; // echoing JSON response echo json_encode($response); } else { // no products found $response["success"] = 0; $response["message"] = "No idioms found"; // echo no users JSON echo json_encode($response); } ?>
When the page is called directly from the browser, this is the JSON formatted data you will see.
http://172.16.16.183/idiomjson/search.php?keyword=baik%20budi {"idioms":[ {"id":"106", "entry":"Baik budi ", "meaning":"Bersikap baik dan penyayang (suka menolong). "}, {"id":"3401", "entry":"Yang merah saga, yang kurik kundi, yang indah bahasa, yang baik budi. ", "meaning":"Rupa yang elok sahaja, tidak disertakan budi bahasa akan tetap menjadi hina di kalangan masyarakat. "} ],"success":1}
STEP 2: CREATE A NEW PROJECT IN ECLIPSE-ADT (Android client-side)
The UI consist of an EditText with id txtkeyword, and a Button id btnsearch.
This is the UI XML file
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".Search" > <TableLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentLeft="true" android:layout_alignParentRight="true" android:layout_alignParentTop="true" > <TableRow android:id="@+id/tableRow1" android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Search for Idiom" android:textAppearance="?android:attr/textAppearanceLarge" /> </TableRow> <TableRow android:id="@+id/tableRow2" android:layout_width="wrap_content" android:layout_height="wrap_content" > <EditText android:id="@+id/txtkeyword" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:ems="10" > <requestFocus /> </EditText> </TableRow> <TableRow android:id="@+id/tableRow3" android:layout_width="wrap_content" android:layout_height="wrap_content" > <Button android:id="@+id/btnsearch" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="Search" /> </TableRow> <TableRow android:id="@+id/tableRow4" android:layout_width="wrap_content" android:layout_height="wrap_content" > </TableRow> </TableLayout> </RelativeLayout>
Search.java
This is only has the UI of the keyword entry. It will be calling another page/screen which will list the idioms that match the search keyword.
package net.kerul.searchjson;//change this to ur package name import android.os.Bundle; import android.app.Activity; import android.content.Intent; import android.view.Menu; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; public class Search extends Activity implements OnClickListener { private EditText txtkeyword; private Button btnsearch; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_search); //link to UI txtkeyword=(EditText)findViewById(R.id.txtkeyword); btnsearch=(Button)findViewById(R.id.btnsearch); btnsearch.setOnClickListener(this); } @Override public void onClick(View v) { if(v.getId()==R.id.btnsearch){ Intent listIntent = new Intent(this, ListResult.class); //send the keyword to the next screen searchIntent.putExtra("key",txtkeyword.getText().toString()); //call the screen for listing startActivity(listIntent); } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.search, menu); return true; } }
Create the ListView named ListResult activity to handle the result listing.
To create a new activity, right click on project and choose New –> Other…
Use the name ListResult for the new activity.
inside this UI XML file copy paste the following XML code. It must have ListView with the id named list.
activity_list_result.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".ListResult" > <!-- Main ListView Always give id value as list(@android:id/list) --> <ListView android:id="@android:id/list" android:layout_width="fill_parent" android:layout_height="wrap_content"/> </RelativeLayout>
Then add another XML file in the layout folder, by right clicking on the layout folder->New->Android XML File. This is to show the record. It will be reiterated for all records successfully fetched. It must be named list_view.xml; it will be called by activity_list_result.xml to populate the idiom data.
list_view.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical" > <!-- Idioms id will be HIDDEN - used to pass to other activity --> <TextView android:id="@+id/id" android:layout_width="fill_parent" android:layout_height="wrap_content" android:visibility="gone" /> <!-- Entry data display --> <TextView android:id="@+id/entry" android:layout_width="fill_parent" android:layout_height="wrap_content" android:textSize="17dip" android:textStyle="bold" /> <!-- the Meaning data display --> <TextView android:id="@+id/meaning" android:layout_width="fill_parent" android:layout_height="wrap_content" android:textSize="14dip" /> </LinearLayout>
and the ListResult.java file to do the fetch record and display processes.
ListResult.java
package net.kerul.searchjson;//change to your package name import java.util.ArrayList; import java.util.HashMap; import java.util.List; import org.apache.http.NameValuePair; import org.apache.http.message.BasicNameValuePair; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import android.app.ListActivity; import android.app.ProgressDialog; import android.content.Intent; import android.os.AsyncTask; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ListAdapter; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.TextView; import android.widget.Toast; public class ListResult extends ListActivity { // Progress Dialog private ProgressDialog pDialog; // Creating JSON Parser object JSONParser jParser = new JSONParser(); ArrayList<HashMap<String, String>> idiomsList; // url to get the idiom list private static String url_search = "http://172.16.16.183/idiomjson/search.php"; // JSON Node names private static final String TAG_SUCCESS = "success"; private static final String TAG_IDIOMS = "idioms"; private static final String TAG_ID = "id"; private static final String TAG_ENTRY = "entry"; private static final String TAG_MEANING = "meaning"; // products JSONArray JSONArray idioms = null; //search key value public String searchkey; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_list_result); Intent myIntent = getIntent(); // gets the arguments from previously created intent searchkey = myIntent.getStringExtra("keyword"); // Hashmap for ListView idiomsList = new ArrayList<HashMap<String, String>>(); // Loading idioms in Background Thread new LoadIdioms().execute(); // Get listview ListView lv = getListView(); // on seleting single idioms // to do something lv.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { // getting values from selected ListItem String iid = ((TextView) view.findViewById(R.id.id)).getText() .toString(); } }); } /** * Background Async Task to Load Idioms by making HTTP Request * */ class LoadIdioms extends AsyncTask<String, String, String> { /** * Before starting background thread Show Progress Dialog * */ @Override protected void onPreExecute() { super.onPreExecute(); pDialog = new ProgressDialog(ListResult.this); pDialog.setMessage("Loading IDIOMS. Please wait..."); pDialog.setIndeterminate(false); pDialog.setCancelable(false); pDialog.show(); } /** * getting Idioms from url * */ protected String doInBackground(String... args) { // Building Parameters List<NameValuePair> params = new ArrayList<NameValuePair>(); //value captured from previous intent params.add(new BasicNameValuePair("keyword", searchkey)); // getting JSON string from URL JSONObject json = jParser.makeHttpRequest(url_search, "GET", params); // Check your log cat for JSON response Log.d("Search idioms: ", json.toString()); try { // Checking for SUCCESS TAG int success = json.getInt(TAG_SUCCESS); if (success == 1) { // products found // Getting Array of Products idioms = json.getJSONArray(TAG_IDIOMS); // looping through All Products for (int i = 0; i < idioms.length(); i++) { JSONObject c = idioms.getJSONObject(i); // Storing each json item in variable String id = c.getString(TAG_ID); String entry = c.getString(TAG_ENTRY); String meaning = c.getString(TAG_MEANING); // creating new HashMap HashMap<String, String> map = new HashMap<String, String>(); // adding each child node to HashMap key => value map.put(TAG_ID, id); map.put(TAG_ENTRY, entry); map.put(TAG_MEANING, meaning); // adding HashList to ArrayList idiomsList.add(map); } } else { // no idioms found //do something } } catch (JSONException e) { e.printStackTrace(); } //return "success"; return null; } /** * After completing background task Dismiss the progress dialog * **/ protected void onPostExecute(String file_url) { // dismiss the dialog after getting the related idioms pDialog.dismiss(); // updating UI from Background Thread runOnUiThread(new Runnable() { public void run() { /** * Updating parsed JSON data into ListView * */ ListAdapter adapter = new SimpleAdapter( ListResult.this, idiomsList, R.layout.list_item, new String[] { TAG_ID, TAG_ENTRY, TAG_MEANING}, new int[] { R.id.id, R.id.entry, R.id.meaning}); // updating listview setListAdapter(adapter); } }); } } }
AND don’t forget the JSONParser.java file. Right click on the package name –>New->Class
JSONParser.java
package net.kerul.searchjson;//change to your package name import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.UnsupportedEncodingException; import java.util.List; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.NameValuePair; import org.apache.http.client.ClientProtocolException; import org.apache.http.client.entity.UrlEncodedFormEntity; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.client.utils.URLEncodedUtils; import org.apache.http.impl.client.DefaultHttpClient; import org.json.JSONException; import org.json.JSONObject; import android.util.Log; public class JSONParser { static InputStream is = null; static JSONObject jObj = null; static String json = ""; // constructor public JSONParser() { } // function get json from url // by making HTTP POST or GET mehtod public JSONObject makeHttpRequest(String url, String method, List<NameValuePair> params) { // Making HTTP request try { // check for request method if(method == "POST"){ // request method is POST // defaultHttpClient DefaultHttpClient httpClient = new DefaultHttpClient(); HttpPost httpPost = new HttpPost(url); httpPost.setEntity(new UrlEncodedFormEntity(params)); HttpResponse httpResponse = httpClient.execute(httpPost); HttpEntity httpEntity = httpResponse.getEntity(); is = httpEntity.getContent(); }else if(method == "GET"){ // request method is GET DefaultHttpClient httpClient = new DefaultHttpClient(); String paramString = URLEncodedUtils.format(params, "utf-8"); url += "?" + paramString; HttpGet httpGet = new HttpGet(url); HttpResponse httpResponse = httpClient.execute(httpGet); HttpEntity httpEntity = httpResponse.getEntity(); is = httpEntity.getContent(); } } catch (UnsupportedEncodingException e) { e.printStackTrace(); } catch (ClientProtocolException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } try { BufferedReader reader = new BufferedReader(new InputStreamReader( is, "iso-8859-1"), 8); StringBuilder sb = new StringBuilder(); String line = null; while ((line = reader.readLine()) != null) { sb.append(line + "\n"); } is.close(); json = sb.toString(); } catch (Exception e) { Log.e("Buffer Error", "Error converting result " + e.toString()); } // try parse the string to a JSON object try { jObj = new JSONObject(json); } catch (JSONException e) { Log.e("JSON Parser", "Error parsing data " + e.toString()); } // return JSON String return jObj; } }
AndroidManifest.xml – don’t forget to add INTERNET Uses Permission. And save the file.
Run on your device/emulator… All the best
.
NEXT - Android Studio 2.2 Training - is available here bit.ly/androidjsk
Comments
Post a Comment