(Pic Source: http://download.oracle.com/docs/cd/B14099_19/web.1012/b15901/sessions008.htm)
So, what is SESSION?
Session in term of server-side scripting concern is the connection between a client (browser) and the server that hosting the web application. Each time a client connects to a web server, there is a connection happens. The connection can be registered in the server and there are a lot of information regarding the connection that can be recorded in the web server. For example the web browser being used or the IP number.
Starting a session
Each unique connection provides a unique session id. To enable the session management of a page, first the session_start() function must be executed. This function can only work if there is no information from the page was sent to the client, not even a space or a single bit.
For example, try the following code.
<html>
<head>
<title>Session Testing</title>
</head>
<body>
<?php
session_start();
//trying to start session management
?>
The session management in this page won't work, because there are a lot of characters has been sent to the client before the session_start() is executed.
</body>
</html>
Output of the script.
This example also doesn’t work. The problem is caused by only a newline (‘\n’) character before the session_start() function.
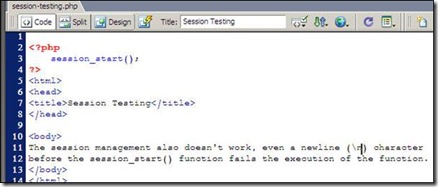
Output of the script.
At last, a working example.
The code;
<?php
session_start();
?>
<html>
<head>
<title>Session Testing</title>
</head>
<body>
The session management WORKS!<br>
<?php
echo "The session id: ".session_id();
?>
</body>
</html>
The output of the script.
So where is this session information is stored?
Currently we are using XAMPP. By default the Apache web server store the session information in the tmp directory. Each session will generate a file with the sess_ as the prefix, and followed by the session id.
Try to open the file and you will nothing is stored in the file where the latest session was created using the script in previous page. This is because there is no session variable is registered.
Session variable registration need to be done in order to store values to be used as long as the session (or the connection) is established. For example, in a web application there are few files connected to each other. In order to maintain a certain amount of value to be used for every pages in the system, we need to store it in the session variable. For example the username of the user who are using the system.
Registering a session variable
To store the username (so that all the pages in the system will be displaying the same username) we need to register a session variable. The following script is to register few session variable.
<?php session_start(); ?>
<html>
<head>
<title>Session Registration</title>
</head>
<body>
This page is to register user's information.<br>
<?php
if(!isset($_SESSION['sessionid'])){
$_SESSION['sessionid']=session_id();//session id
$_SESSION['browser']=$_SERVER['HTTP_USER_AGENT'];//browser
$_SESSION['ipnumber']=$_SERVER['REMOTE_ADDR'];//client's ip
$_SESSION['username']="kerul";//the username
$_SESSION['name']="Khirulnizam Abd Rahman";//full name
$_SESSION['level']=1;//user access level
}
echo "Session id: ".$_SESSION['sessionid']."<br>";
echo "User browser: ".$_SESSION['browser']."<br>";
echo "Client IP: ".$_SESSION['ipnumber']."<br>";
?>
</body>
</html>
The script provide the registration of four session variable namely; sessionid, username, name and level, with their respective value.
Now open the file where the server store the session id in notepad.
This is the content of the session file.
Checking the session variable.
<?php
session_start();
?>
<html>
<head>
<title>Session Checking</title>
</head>
<body>
This page is to check whether user's information are stored in the session.<br>
<?php
if (isset($_SESSION['sessionid'])){
echo $_SESSION['sessionid'] .'<br>';
echo $_SESSION['browser'].'<br>';
echo $_SESSION['ipnumber'].'<br>';
echo $_SESSION['username'] .'<br>';
echo $_SESSION['name'] .'<br>';
echo $_SESSION['level'] .'<br>';
}
else{
$_SESSION['sessionid']=session_id();
$_SESSION['browser']=$_SERVER['HTTP_USER_AGENT'];
$_SESSION['ipnumber']=$_SERVER['REMOTE_ADDR'];
$_SESSION['username']="kerul";
$_SESSION['name']="Khirulnizam Abd Rahman";
$_SESSION['level']=1;
}
?>
</body>
</html>
Using the session variable.
<?php session_start(); ?>
<html>
<head>
<title>Session Usage</title>
</head>
<body>
This page is to use user's information stored in session.<br>
<?php
echo $_SESSION['sessionid'].'<br>';
echo $_SESSION['browser'].'<br>';
echo $_SESSION['ipnumber'].'<br>';
echo $_SESSION['username'] .'<br>';
echo $_SESSION['name'] .'<br>';
echo $_SESSION['level'] .'<br>';
?>
</body>
</html>
<?php
session_start();
?>
<html>
<head>
<title>Session Destroy</title>
</head>
<body>
This page is to destroy a session.<br>
<?php
if (isset($_SESSION['sessionid'])){
session_destroy();//this to destroy all session info
}
?>
</body>
</html>
SIMPLE EXAMPLE
Protect ur Treasure App!
This simple application is to illustrate the usage of server session. You have two web pages; a page that contains your ‘treasure’, and another page which is the guardian of the ‘treasure’. In order for the right user to acquire your ‘treasure’, the person need to provide the right username and password to the guardian (login page).
1st page – the login page (guardian) – session registration.
File name: guardian.php
<?php session_start() ?>
<html>
<head>
<title>Session Register</title>
</head>
<body>
I'm the guardian!<br>
<img src="guardian.jpg"><br>
Provide username and password to discover the treasure!<br>
<form method='GET' action="">
Username <input type="text" name="username"><br>
Password <input type="password" name="psword"><br>
Password <input type="submit" value="Unlock"><br>
</form>
<hr>
Message from the Guardian!<br>
<?php
$usrname=$_GET['username'];
$usrpswd=$_GET['psword'];
//guardian password
$guard_username="kerul";
$guard_password="kerul.net";
if ($usrname==NULL || $usrpswd==NULL){
echo "Provide the information, human!<br>";
}
else{
if($usrname==$guard_username && $usrpswd==$guard_password){
//allowed to enter
echo "<a href='treasure.php'>Click to get ur treasure</a><br>";
//set the session
if(!isset($_SESSION['sessionid'])){
$_SESSION['sessionid']=session_id();//session id
$_SESSION['username']=$usrname;//the username
}
}
else{
echo "You have no right to the treasure, <br>";
echo "you might wanna try again!";
}
}
?>
</body>
</html>
2nd page – the treasure – session checking.
File name: treasure.php
<?php
//this script is to check session to verify user login
session_start();
if(!isset($_SESSION["username"])){ //if session NOT set
echo "You are not authorised, human".
"<a href='guardian.php'>Click here to login.</a>";
exit(0);
}
?>
<html>
<head>
<title>Session Checking and Usage</title>
</head>
<body>
<?php
echo "You deserve the treasure,".$_SESSION['username']."!<br>";
?>
<img src="treasure.jpg"><br>
Once finished, <a href="lock.php">LOCK the treasure back!</a>
</body>
</html>
3rd page – logout - session destroyer.
File name: lock.php
<?php
session_start();
?>
<html>
<head>
<title>Session Destroy</title>
</head>
<body>
<?php
if (isset($_SESSION['username'])){
session_destroy();//this to destroy all session info
}
?>
The treasure has been LOCKED. <br>
<a href="guardian.php">Meet the guardian to UNLOCK!</a><br>
</body>
</html>
Download codes here –>
Wah.. guru PHP lagi power!
ReplyDeleteAs u said b4, human got no power, power comes from the human creator...
ReplyDelete